How To Have Your Ar Camera Move In Unity
In this tutorial, nosotros are going to learn how we can make the camera follow an object in Unity3D using two different methods. The first one is by writing a script that updates the position of the camera according to position of the object. The second one is using Cinemachine component of Unity3D.
A Niggling Chip Vector Algebra
Earlier we outset writing our CameraFollow script, outset we demand to review our noesis about vectors a niggling fleck. If y'all are already familiar with vector algebra, you may skip this function. As you probably know, we stand for positions of objects in Unity3D using vectors. In second space, we need 2 numbers to represent a position. Too, for 3D space, we need 3 numbers. To make information technology unproblematic, for now, we are going to study in 2D space showtime, so move our discussion to 3D space.
As we mentioned to a higher place, in 2D infinite we need 2 numbers to represent a position. For instance, A=(2,3) represents a indicate on 2D space such that 2 units on the x-axis, and iii units on the y-centrality. In the aforementioned way, B=(ii,-ane) represents another vector.
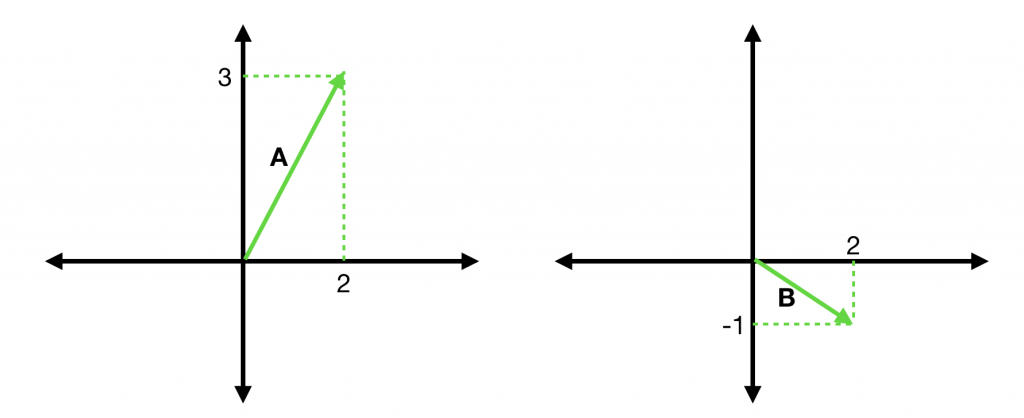
Vectors are cool mathematical objects that you can perform several mathematical operations like summation, subtraction, different kind of multiplications, etc. If you would like to deep dive into game evolution, you should definitely exist comfy if you are not familiar with them. But for this tutorial, nosotros are simply going to embrace bones operations.
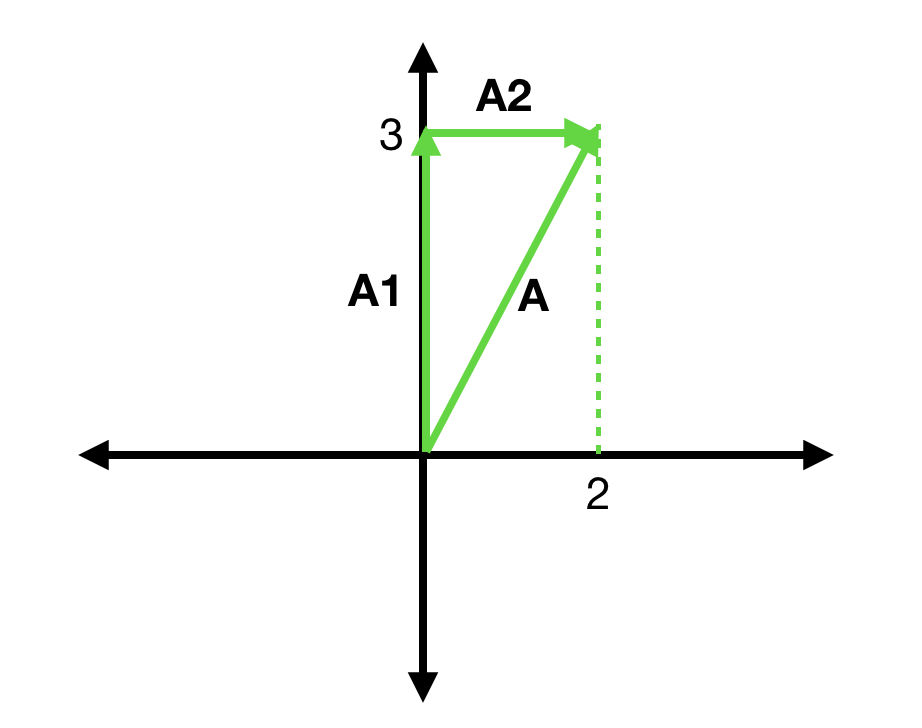
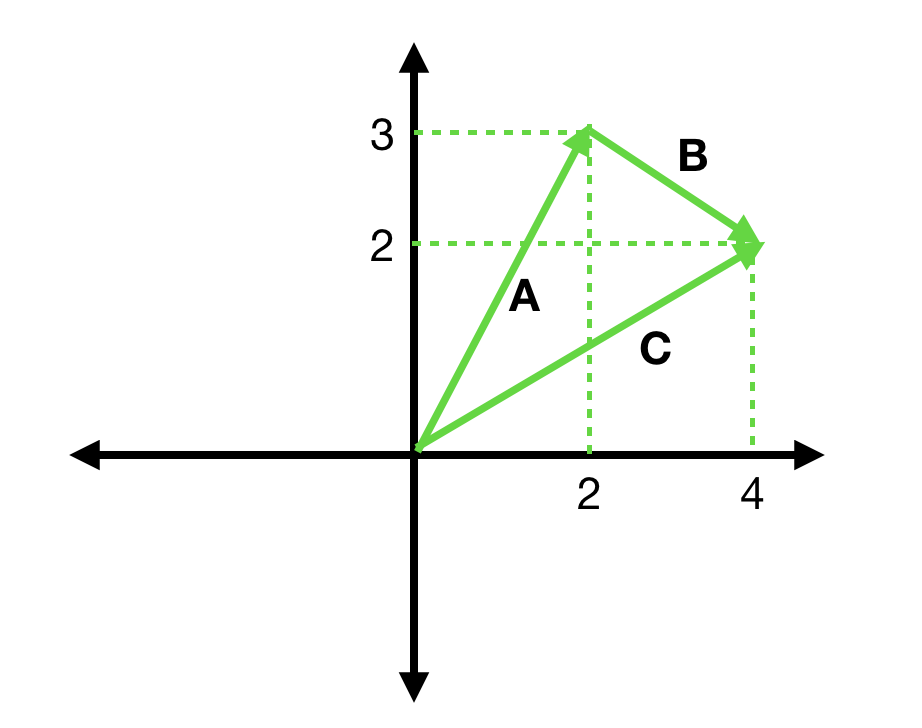
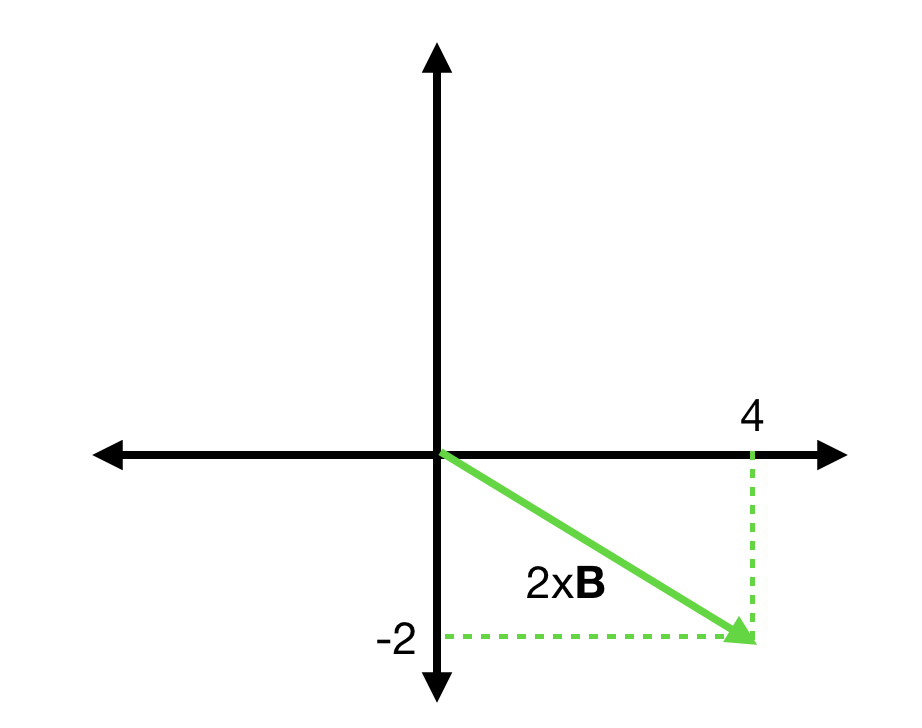
Presume that you are at the center of the coordinate space. This point is chosen as the origin. And the coordinates of the origin are (0,0) in 2D space. Now assume that you are walking on the vertical axis to the up direction( i.e y-axis) past three units then in the right direction by 2 units. Your terminal position will be the point (ii,3). Alternatively, you tin walk forth the A. The final position will be the aforementioned. Permit's say A1=(0,3) and A2=(2,0). As yous may discover we tin stand for vector A equally the summation of two vectors, A1+A2. When we want to sum two vectors, nosotros sum the first components and obtain the get-go component of the final vector. Also, we have to add the second component of ii vectors to obtain the 2d component of the concluding vector.
Similarly, we can add any two vectors to obtain a third vector. For instance, if we add together vector B to vector A, we get C which represents some other position in the 2d space.
In addition to summation, nosotros tin multiply vectors by numbers (furthermore, you can multiply a vector with another vector but this is out of our interest, here). For case, we can multiply vector B by 2. In this case, we will become a vector that is represented as (4,-two). Furthermore, you can multiply a vector with a negative number. For example, if you multiply B with -i, then you volition get (-2,1).
All of our word here can be generalized to higher dimensions. All the mathematics is the aforementioned for 3D space as well.
Up to now, we made a review of the basics of vector algebra and we are going to use these operations while we are writing a camera follow script.
Post-obit an Object using CameraFollow Script
First, we will showtime with a bones script then we volition make information technology ameliorate. Let'south start.
Assume that we have an object at some position in our scene. Permit's say the position vector that represents its position is O. And we have a camera that is located at some point in the scene that is represented by a position vector C.
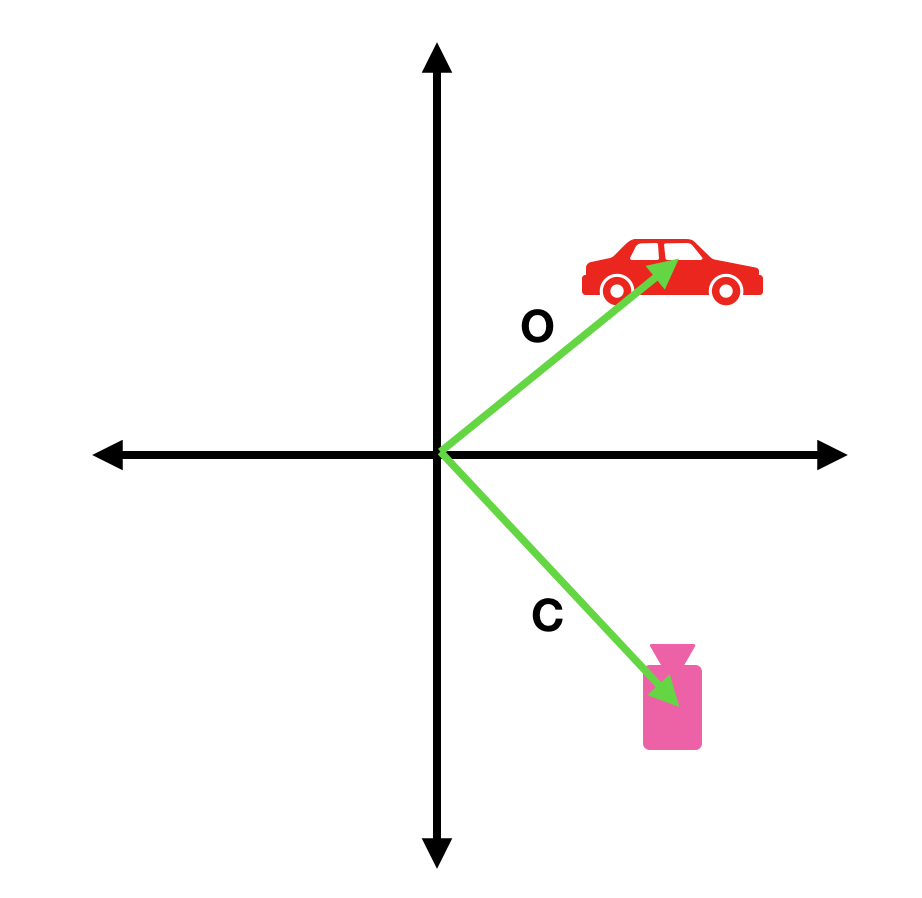
Our aim is to update the position of the photographic camera according to the object's position. Therefore, nosotros take to decide the position of the camera according to the object's position. To do this, nosotros need the position vector from the object to the camera. We can obtain this relative position vector by subtracting O from C:
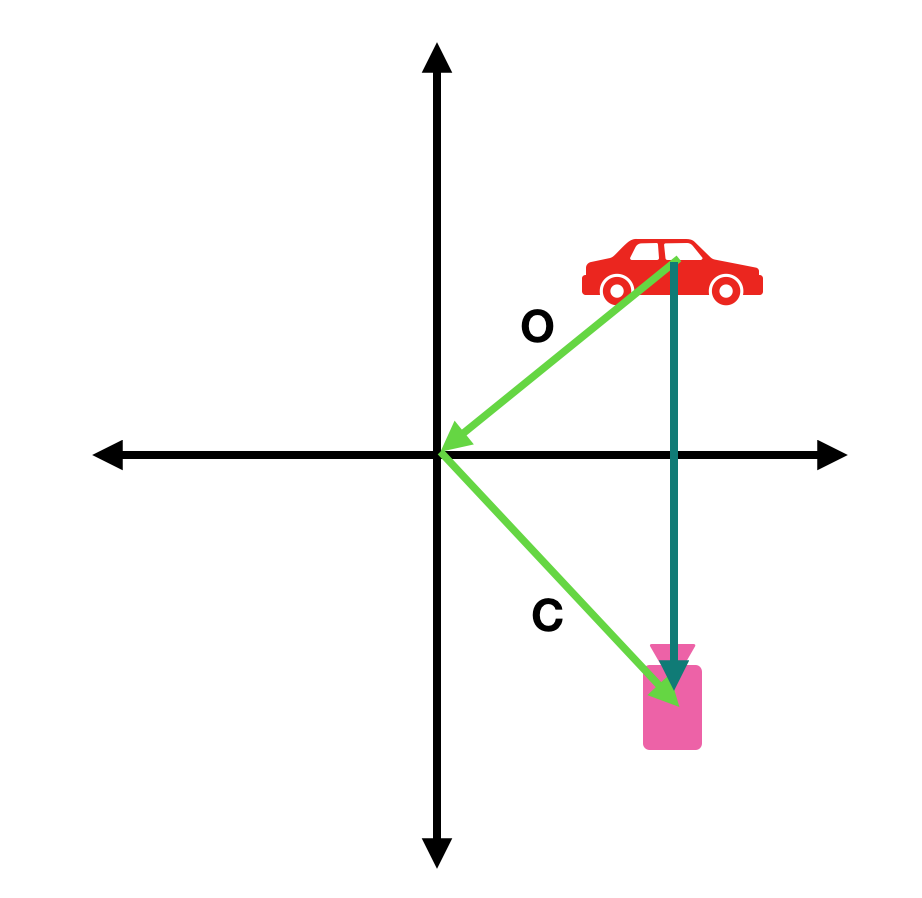
Therefore, we tin determine the new position of the photographic camera past adding this relative direction vector to the object'southward instantaneous position.
Create a new script and change its name to CameraFollow. Assign this script to your primary camera.
using Organization.Collections; using System.Collections.Generic; using UnityEngine; public class CameraFollow : MonoBehaviour { public Transform targetObject; individual Vector3 initalOffset; individual Vector3 cameraPosition; void Start( ) { initalOffset = transform.position - targetObject.position; } void FixedUpdate( ) { cameraPosition = targetObject.position + initalOffset; transform.position = cameraPosition; } }
As mentioned above, we get the relative position vector by subtracting the target object'south initial position from the camera's initial position. We add this commencement vector to the object's position vector to find the new position vector of the photographic camera. Do not forget to assign the target object into your script from the inspector.
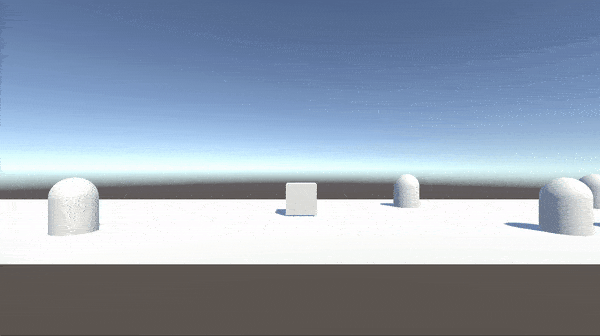
We tin can make it smoother if we use the Vector3.Lerp method. Lerp method is used for linear interpolation. If we update the position of the camera with a delay, we volition obtain a smooth move. If you add a smoothness variable, you can control the filibuster.
public course CameraFollow : MonoBehaviour { public float smoothness; public Transform targetObject; private Vector3 initalOffset; private Vector3 cameraPosition; void Beginning( ) { initalOffset = transform.position - targetObject.position; } void FixedUpdate( ) { cameraPosition = targetObject.position + initalOffset; transform.position = Vector3.Lerp(transform.position, cameraPosition, smoothness*Time.fixedDeltaTime) ; } }
Let me explain linear interpolation for those who are not familiar with. Linear interpolation is a method that returns a value betwixt two values. In the Vector3.Lerp method, we used 3 arguments. The showtime and 2nd ones are position vectors and the third one is the interpolation value. Assume that the first vector is a aught vector and the second vector is (1,0,0). If the tertiary value is 0.five then Vector3.Lerp method will render a vector (0.5, 0, 0). Likewise, in our CameraFollow script, we used smoothness*Fourth dimension.fixedDeltaTime. Therefore, in each FixedUpdate() method phone call, the position vector is updated a small amount. This volition create a smooth move.
And this is the issue:
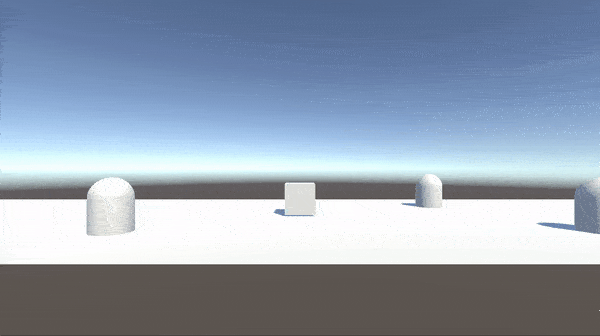
Let's take our script a step further. At present, nosotros would similar to specify a few coordinates relative to the target object and switch the position of the photographic camera to these specified coordinates.
To practise this, we can declare an enum and therefore when we want to switch the relative position, we can specify ane of the selections.
public class CameraFollow : MonoBehaviour { public float smoothness; public Transform targetObject; private Vector3 initalOffset; private Vector3 cameraPosition; public enum RelativePosition { InitalPosition, Position1, Position2 } public RelativePosition relativePosition; public Vector3 position1; public Vector3 position2; void Start( ) { relativePosition = RelativePosition.InitalPosition; initalOffset = transform.position - targetObject.position; } void FixedUpdate( ) { cameraPosition = targetObject.position + CameraOffset(relativePosition) ; transform.position = Vector3.Lerp(transform.position, cameraPosition, smoothness*Time.fixedDeltaTime) ; transform.LookAt(targetObject) ; } Vector3 CameraOffset(RelativePosition ralativePos) { Vector3 currentOffset; switch (ralativePos) { case RelativePosition.Position1: currentOffset = position1; interruption ; example RelativePosition.Position2: currentOffset = position2; suspension ; default : currentOffset = initalOffset; break ; } return currentOffset; } }
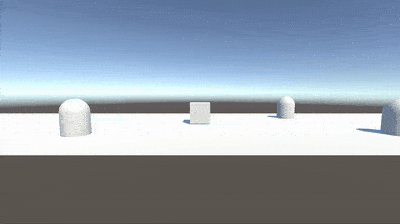
Post-obit an Object Using Cinemachine
In the second office of this tutorial, we are going to see how we tin follow an object using the cinemachine component. Starting time, nosotros need to download and import the cinemachine from the asset store. If you take problems with importing cinemachine, yous may need to restart Unity.
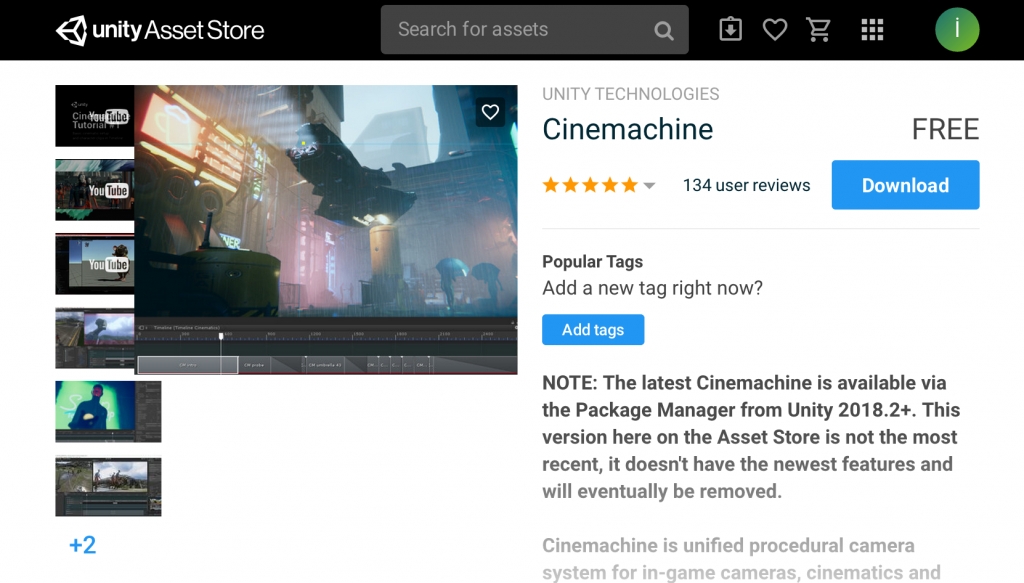
Add the Cinemachine Encephalon component to your primary camera. Cinemachine Encephalon is the control signal for the virtual cameras that we will create.
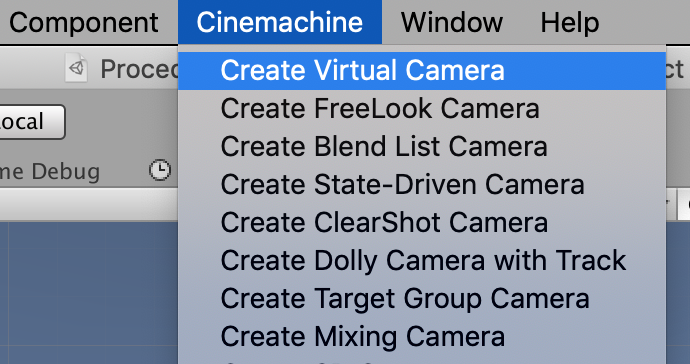
At present nosotros can create several virtual cameras, command the behaviors of them and switching between them. But for this tutorial, nosotros volition create simply one virtual photographic camera to show how we can make the photographic camera follow an object.
Create a virtual camera from the Cinemachine menu.
Click on the newly created virtual camera. Accommodate your camera position, rotation or other settings every bit if information technology is your primary camera. Then assign your target object to the Follow slot. If you would similar to rotate your photographic camera according to your object, you can assign information technology to Look At slot, too.
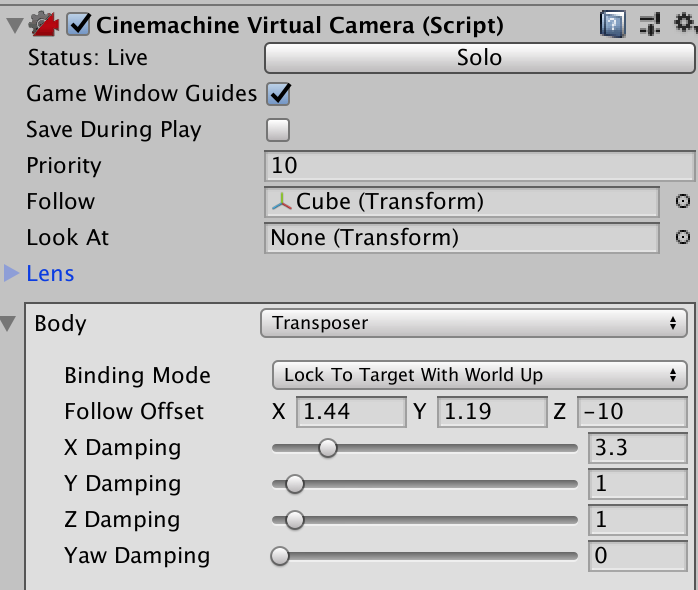
You tin fine-tune the delay of the photographic camera behind the motion of the object by changing damping values in each direction.
Source: https://www.codinblack.com/how-to-make-the-camera-follow-an-object-in-unity3d/
Posted by: bairdanowbod.blogspot.com
0 Response to "How To Have Your Ar Camera Move In Unity"
Post a Comment